jQuery专题
Abstract Keywords Js 专题 jQuery 专题
Citation Yao Qing-sheng.jQuery专题.FUTURE & CIVILIZATION Natural/Social Philosophy & Infomation Sciences,20210327. https://yaoqs.github.io/20210327/jquery-zhuan-ti/
[TOC]
※菜鸟教程
※常用
- 基本选择器
1 | $("#id") //ID选择器 |
- 层次选择器
1 | $("#id>.classname ") //子元素选择器 |
- 过滤选择器(重点)
1 | $("li:first") //第一个li |
- 内容过滤选择器
1 | $("div:contains('Runob')") // 包含 Runob文本的元素 |
- 可见性过滤选择器
1 | $("li:hidden") //匹配所有不可见元素,或type为hidden的元素 |
- 属性过滤选择器
1 | $("div[id]") //所有含有 id 属性的 div 元素 |
- 状态过滤选择器
1 | $("input:enabled") // 匹配可用的 input |
- 表单选择器
1 | $(":input") //匹配所有 input, textarea, select 和 button 元素 |
※JS/Jquery 表单方式提交总结
- submit提交
(1). submit 按钮式提交
缺点:在提交前不可修改提交的form表单数据
1 | // 1. html |
(2). onsubmit方式提交
优点:在请求提交操作(action)时,可对提交的数据进行处理
1 | // 1. html |
1 | // 2.js |
- formData 提交
1 | // 1. html |
1 | // 2. js |
- 动态添加表单提交, js方式提交
- 动态追加的form表单
1
2
3
4
5
6var exportForm = $('<form action="'+ajaxUrl+'" method="post">\
<input type="hidden" name="beginDate" value="'+$(".beginDate").val()+'"/>\
</form>');
$(document.body).append(exportForm);
exportForm.submit(); // 表单提交
exportForm.remove();- 静态form表单,js方式提交
1
2
3
4// 1. html
<form action="'+ajaxUrl+'" method="post">
<input type="hidden" name="beginDate" value="'+$(".beginDate").val()+'"/>
</form>1
2
3// 2. js/JQuery
document.getElementById("form").submit(); // js写法
$("#form").submit(); // jquery写法
※jQuery 常用代码集锦
选择或者不选页面上全部复选框
1
2
3
4
5var tog = false; // or true if they are checked on load
$('a').click(function() {
$("input[type=checkbox]").attr("checked",!tog);
tog = !tog;
});取得鼠标的X和Y坐标
1
2
3
4
5
6$(document).mousemove(function(e){
$(document).ready(function() {
$().mousemove(function(e){
$('#XY').html("Gbin1 X Axis : " + e.pageX + " | Gbin1 Y Axis " + e.pageY);
});
});判断一个图片是否加载完全
1
2
3$('#theGBin1Image').attr('src', 'image.jpg').load(function() {
alert('This Image Has Been Loaded');
});判断cookie是否激活或者关闭
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15var dt = new Date();
dt.setSeconds(dt.getSeconds() + 60);
document.cookie = "cookietest=1; expires=" + dt.toGMTString();
var cookiesEnabled = document.cookie.indexOf("cookietest=") != -1;
if(!cookiesEnabled)
{
//cookies have not been enabled
}
+ 强制过期cookie
```js
var date = new Date();
date.setTime(date.getTime() + (x * 60 * 1000));
$.cookie('example', 'foo', { expires: date });在表单中禁用“回车键”,表单的操作中需要防止用户意外的提交表单
1
2
3
4
5$("#form").keypress(function(e) {
if (e.which == 13) {
return false;
}
});清除所有的表单数据
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20function clearForm(form) {
// iterate over all of the inputs for the form
// element that was passed in
$(':input', form).each(function() {
var type = this.type;
var tag = this.tagName.toLowerCase(); // normalize case
// it's ok to reset the value attr of text inputs,
// password inputs, and textareas
if (type == 'text' || type == 'password' || tag == 'textarea')
this.value = "";
// checkboxes and radios need to have their checked state cleared
// but should *not* have their 'value' changed
else if (type == 'checkbox' || type == 'radio')
this.checked = false;
// select elements need to have their 'selectedIndex' property set to -1
// (this works for both single and multiple select elements)
else if (tag == 'select')
this.selectedIndex = -1;
});
};禁止多次递交表单
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15$(document).ready(function() {
$('form').submit(function() {
if(typeof jQuery.data(this, "disabledOnSubmit") == 'undefined') {
jQuery.data(this, "disabledOnSubmit", { submited: true });
$('input[type=submit], input[type=button]', this).each(function() {
$(this).attr("disabled", "disabled");
});
return true;
}
else
{
return false;
}
});
});自动将数据导入selectbox中
1
2
3
4
5
6
7
8
9
10
11$(function(){
$("select#ctlJob").change(function(){
$.getJSON("/select.php",{id: $(this).val(), ajax: 'true'}, function(j){
var options = '';
for (var i = 0; i < j.length; i++) {
options += '<option value="' + j[i].optionValue + '">' + j[i].optionDisplay + '</option>';
}
$("select#ctlPerson").html(options);
})
})
})创建一个嵌套的过滤器
1
.filter(":not(:has(.selected))") //去掉所有不包含class为.selected的元素
使用has()来判断一个元素是否包含特定的class或者元素
1
2
3
4//jQuery 1.4.* includes support for the has method. This method will find
//if a an element contains a certain other element class or whatever it is
//you are looking for and do anything you want to them.
$("input").has(".email").addClass("email_icon");使用jQuery切换样式
1
2//Look for the media-type you wish to switch then set the href to your new style sheet
$('link[media='screen']').attr('href', 'Alternative.css');如何正确使用ToggleClass
1 | //Toggle class allows you to add or remove a class |
使用jQuery来替换一个元素
1
$('#thatdiv').replaceWith('fnuh');
绑定一个函数到一个事件
1
2
3$('#foo').bind('click', function() {
alert('User clicked on "foo."');
});使用jQuery预加载图片
1
2jQuery.preloadImages = function() { for(var i = 0; i').attr('src', arguments[i]); } };
// Usage $.preloadImages('image1.gif', '/path/to/image2.png', 'some/image3.jpg');设置任何匹配一个选择器的事件处理程序
1
2
3
4
5
6
7
8
9
10
11
12
13
14$('button.someClass').live('click', someFunction);
//Note that in jQuery 1.4.2, the delegate and undelegate options have been
//introduced to replace live as they offer better support for context
//For example, in terms of a table where before you would use..
// .live()
$("table").each(function(){
$("td", this).live("hover", function(){
$(this).toggleClass("hover");
});
});
//Now use..
$("table").delegate("td", "hover", function(){
$(this).toggleClass("hover");
});自动的滚动到页面特定区域
1
2
3
4
5
6
7
8jQuery.fn.autoscroll = function(selector) {
$('html,body').animate(
{scrollTop: $(selector).offset().top},
);
}
//Then to scroll to the class/area you wish to get to like this:
$('.area_name').autoscroll();检测各种浏览器
1
2
3
4Detect Safari (if( $.browser.safari)),
Detect IE6 and over (if ($.browser.msie && $.browser.version > 6 )),
Detect IE6 and below (if ($.browser.msie && $.browser.version <= 6 )),
Detect FireFox 2 and above (if ($.browser.mozilla && $.browser.version >= '1.8' )限制textarea的字符数量
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25jQuery.fn.maxLength = function(max){
this.each(function(){
var type = this.tagName.toLowerCase();
var inputType = this.type? this.type.toLowerCase() : null;
if(type == "input" && inputType == "text" || inputType == "password"){
//Apply the standard maxLength
this.maxLength = max;
}
else if(type == "textarea"){
this.onkeypress = function(e){
var ob = e || event;
var keyCode = ob.keyCode;
var hasSelection = document.selection? document.selection.createRange().text.length > 0 : this.selectionStart != this.selectionEnd;
return !(this.value.length >= max && (keyCode > 50 || keyCode == 32 || keyCode == 0 || keyCode == 13) && !ob.ctrlKey && !ob.altKey && !hasSelection);
};
this.onkeyup = function(){
if(this.value.length > max){
this.value = this.value.substring(0,max);
}
};
}
});
};
//Usage:
$('#gbin1textarea').maxLength(500);使用jQuery克隆元素
1
var cloned = $('#gbin1div').clone();
元素屏幕居中
1
2
3
4
5
6jQuery.fn.center = function () {
this.css('position','absolute');
this.css('top', ( $(window).height() - this.height() ) / +$(window).scrollTop() + 'px');
this.css('left', ( $(window).width() - this.width() ) / 2+$(window).scrollLeft() + 'px');return this;
}
//Use the above function as: $('#gbin1div').center();简单的tab标签切换
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41jQuery('#meeting_tabs ul li').click(function(){
jQuery(this).addClass('tabulous_active').siblings().removeClass('tabulous_active');
jQuery('#tabs_container>.pane:eq('+jQuery(this).index()+')').show().siblings().hide();
})
<div id="meeting_tabs">
<ul>
<li class="tabulous_active"><a href="#" title="">进行中</a></li>
<li><a href="#" title="">未开始</a></li>
<li><a href="#" title="">已结束</a></li>
<li><a href="#" title="">全部</a></li>
</ul>
<div id="tabs_container">
<div class="pane" >1</div>
<div class="pane" >2</div>
<div class="pane" >3</div>
<div class="pane" >4</div>
</div>
</div>
jQuery('#meeting_tabs ul li').click(function(){
jQuery(this).addClass('tabulous_active').siblings().removeClass('tabulous_active');
jQuery('#tabs_container>.pane:eq('+jQuery(this).index()+')').show().siblings().hide();
})
<div id="meeting_tabs">
<ul>
<li class="tabulous_active"><a href="#" title="">进行中</a></li>
<li><a href="#" title="">未开始</a></li>
<li><a href="#" title="">已结束</a></li>
<li><a href="#" title="">全部</a></li>
</ul>
<div id="tabs_container">
<div class="pane" >1</div>
<div class="pane" >2</div>
<div class="pane" >3</div>
<div class="pane" >4</div>
</div>
</div>
※query操作表单元素代码
1
2
3
4
5
6
7
/*假设在一个表单中有一个按钮id="save"*/
$(document).ready(function(){
$("#save").click(function(){
$("#save").attr("disabled",true);//设为不可用
$("#form1")[0].submit();//如果你有很多个id为form1的表单也没关系,只有第一个会提交的哈哈.
});
});
取下拉菜单选中项的文本
1
2$("#select option[selected]").text();//select和option之间有空格,option为select的子元素
$("#select option:selected").text();//如果写成$("#select").text();会把所有下拉菜单的文本选择出来获取和设置下拉菜单的值
1
2$("#select").val();//取值
$("#select").val("value");//设置,如果select中有值为value的选项,该选项就会被选中,如果不存在,则select不做任何变动清空下拉菜单
1
2$("#select").empty();
$("#select").html("");给下列菜单添加元素
1
2$('<option value="1">1</option>').appendto($("#select"));
$("#select").append('<option value="1">1</option>');取单选框值
1
$("#id[checked]").val();
单选或复选按钮的选择
1
2
3
4$("#id[value=val]").attr("checked",true);//选择
$("#id[value=val]").attr("checked","");//取消选择
$("#id[value=val]").attr("checked",false);//取消选择
$("#id[value=val]").removeattr("checked");//取消选择取复选框值
1
2
3
4$("input[type=checkbox][checked]").each(function(){
alert($(this).val());
})
//如果用$("input[type=checkbox][checked]").val(),只会返回第一个被选中的值判断单选或复选框是否被选中
1
2
3if($("#id").attr("checked")){}//判断选中
if($("#id").attr("checked")==true){}//判断选中
if($("#id").attr("checked")==undefined){}//判断未选中元素可用不可用
1
2$("#id").attr("disabled",false);//设为可用
$("#id").attr("disabled",true);//设为不可用判断元素可用不可用
1
2if($("#id").attr("disabled")){}//判断不可用
if($("#id").attr("disabled")==undefined){}//判断可用
文本框操作
1
2
3
4取 值:var textval = $("#text_id").attr("value");
var textval = $("#text_id").val();
清除内容:$("#txt").attr("value","");
填充内容:$("#txt").attr("value",'123');文本域操作
1
2
3
4取 值:var textval = $("#text_id").attr("value");
var textval = $("#text_id").val();
清除内容:$(”#txt”).attr("value","");
填充内容:$(”#txt”).attr("value",'123');单选按钮操作
1
2取 值:var valradio = $("input[@type=radio][@checked]").val(); //只有一组Radio情况下
var valradio =$('input[@name=chart][@checked]').val(); //多组Radio情况下,根据name取一组的值下拉框操作
1
2
3
4取 值:var selectval = $('#sell').val();
设置选中:$("#select_id").attr("value",'test');//设置value=test的项目为当前选中项
添加新项:$("<option value='test'>test</option><option value='test2'>test2</option>").appendTo("#select_id")//添加下拉框的option
清空下拉框:$("#select_id").empty();//清空下拉框多选框操作
1
2
3取 值:$("#chk_id").attr("checked",'');//未选中的值
$("#chk_id").attr("checked",true);//选中的值
if($("#chk_id").attr('checked')==undefined) //判断是否已经选中
※写JQuery插件的基本知识
- 用JQuery写插件时,最核心的方法有如下两个:
1 | $.extend(object) 可以理解为JQuery 添加一个静态方法。 |
基本的定义与调用:
1 | /* $.extend 定义与调用 |
- jQuery(function () { }); 与 (function ($) { })(jQuery);的区别:
1 | jQuery(function () { }); |
jQuery(function () { });是某个DOM元素加载完毕后执行方法里的代码。
(function ($) { })(jQuery); 定义了一个匿名函数,其中jQuery代表这个匿名函数的实参。通常用在JQuery插件开发中,起到了定义插件的私有域的作用。
※jQuery实现手势解锁密码特效
HTML:
1 | <div id="gesturepwd" style="position: absolute;width:440px;height:440px;left:50%;top:50%; |
首次渲染:
1 | $("#gesturepwd").GesturePasswd({ |
密码判断代码:(这里的密码“34569”意思为页面上从上到下,从左到右的9个原点中的5个点)
1 | $("#gesturepwd").on("hasPasswd",function(e,passwd){ |
核心脚本调用展示:
1 | (function ($) { |
※通过jquery的ajax请求本地的json文件方法
- ajax
1 | $(function(){ |
- 还可以通过$.getJSON来获取本地json文件
1 | /* getJSON*/ |
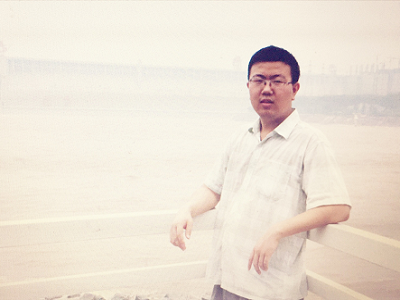
Author: Yao Qing-sheng Blog: https://yaoqs.github.io/ Email: 350788415@qq.com
Address:Department of Natural/Social Philosophy & Infomation Sciences, CHINA
Biography...
Address:Department of Natural/Social Philosophy & Infomation Sciences, CHINA
Biography...
Like this article? Support the author with